Introduction
Python is a versatile and widely-used programming language, known for its simplicity and readability. It offers a multitude of applications, from simple scripting tasks to complex data analysis and web development. One of the most convenient ways to execute Python code is through the bash command line. In this article, we will explore different methods of running Python code from the bash command line, empowering developers to streamline their development workflow.
Why Execute Python from the bash Command Line?
The command line interface (CLI) provides developers with a powerful environment to interact with their code quickly. By running Python from the command line, developers can easily test snippets of code, create scripts for automation, and integrate Python seamlessly with other command-line tools.
Method 1: Running a Python Script
The most straightforward way to execute Python code from the bash command line is by running a Python script. Follow these steps:
- Create a new file with a
.py
extension, e.g.,myscript.py
. - Add your Python code to the script file. For example:
# myscript.py
print("Hello, world!")
- Save the file.
- Open the terminal (bash) and navigate to the directory where your script is located.
- Execute the Python script using the
python
command:
python myscript.py
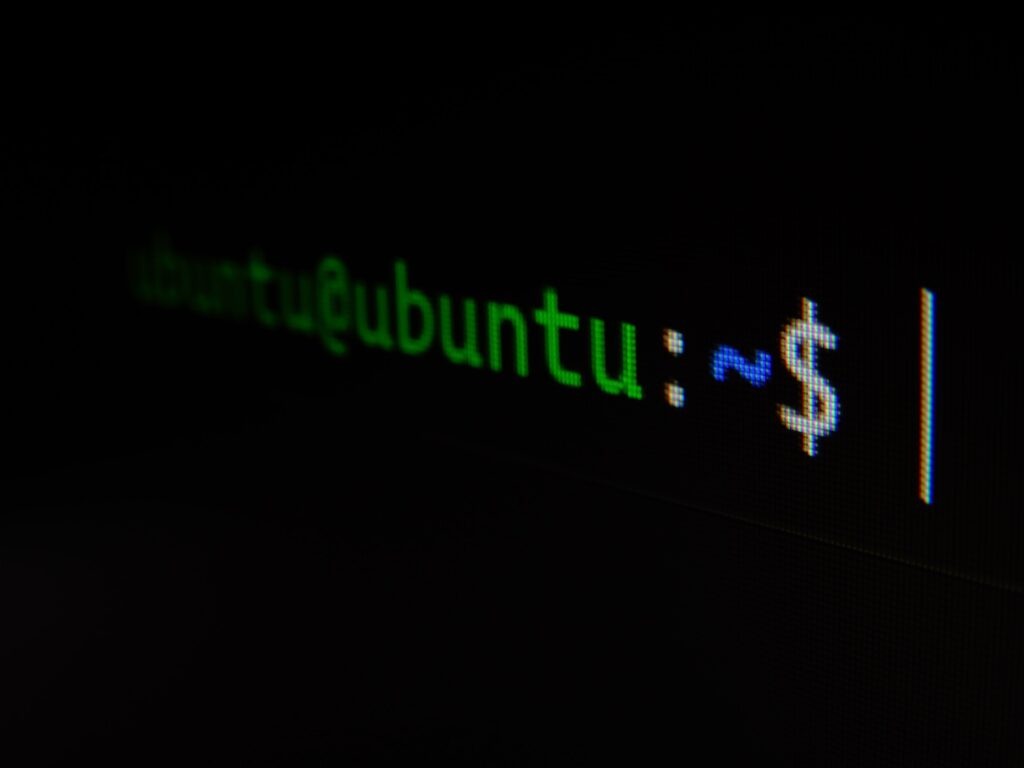
Method 2: Using Shebang (#!/usr/bin/env python)
You can make your Python script executable directly from the command line by adding a shebang at the top of the script. The shebang is a special line that tells the operating system which interpreter should be used to run the script. Here’s how to do it:
- Open your Python script (
myscript.py
). - Add the shebang line as the first line of the script:
#!/usr/bin/env python
print("Hello, world!")
- Save the file.
- Make the script executable using the
chmod
command:
chmod +x myscript.py
- Execute the script directly from the command line:
./myscript.py
Method 3: Using Python 3
In some systems, python
refers to Python 2.x, while python3
refers to Python 3.x. If you have both versions installed, you might need to specify python3
explicitly to run Python 3 code:
python3 myscript.py
This ensures that your script is executed using Python 3, avoiding any compatibility issues.
Method 4: Inline Python Code
Apart from running scripts, you can also execute inline Python code directly from the bash command line using the -c
option. For example:
python -c "print('Hello, world!')"
This command will execute the provided Python code and display the output in the terminal.
Method 5: Passing Command Line Arguments
You can pass command-line arguments to your Python script when executing it from the bash command line. These arguments can be accessed in the script using the sys.argv
list. For example:
code# myscript.py
import sys
print("Hello, " + sys.argv[1] + "!")
To pass arguments, simply add them after the script name:
python myscript.py John
Output:
Hello, John!
Conclusion
Executing Python code from the bash command line provides developers with a flexible and efficient means of interacting with their code. By running Python scripts, using shebangs, or executing inline code, developers can streamline their development workflow and easily test and deploy Python applications. Understanding these methods empowers developers to harness the full potential of Python and the command line interface for their projects.