In the world of programming, developing applications that interact with users is both exciting and challenging. User input, though necessary, can be unpredictable. In Python, accepting user input is a common practice. However, handling user input errors can sometimes lead to unexpected crashes or errors in the program.
Introduction to user input errors:
Let’s explore a scenario where a simple Python program prompts users to input their age and determines whether they are eligible to vote in the United States. The initial version of the program looks like this:
# Note: Python 2.7 users should use `raw_input`, the equivalent of 3.X's `input`
age = int(input("Please enter your age: "))
if age >= 18:
print("You are able to vote in the United States!")
else:
print("You are not able to vote in the United States.")
This program works perfectly when the user enters valid, numeric data. However, the trouble arises when an unexpected input is provided, as seen in the example below:
Please enter your age: dickety six
Traceback (most recent call last):
File "canyouvote.py", line 1, in <module>
age = int(input("Please enter your age: "))
ValueError: invalid literal for int() with base 10: 'dickety six'
Instead of gracefully handling this situation, the program crashes, displaying user input errors messages. To improve this program and create a more user-friendly experience, we can modify it to handle unexpected inputs.
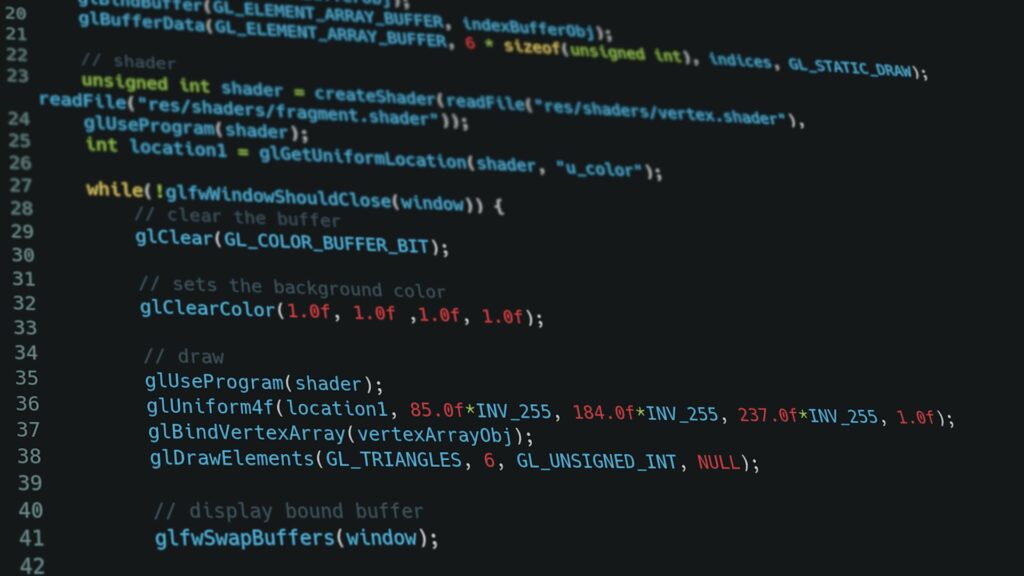
Here’s a revised version that continuously prompts the user until a valid age is entered:
while True:
user_input = input("Please enter your age: ")
try:
age = int(user_input)
if age >= 18:
print("You are able to vote in the United States!")
else:
print("You are not able to vote in the United States.")
break
except ValueError:
print("Sorry, I didn't understand that. Please enter a valid age.")
In this updated version, we introduced a while loop that constantly requests user input. The program attempts to convert the input to an integer within a try-except block. If an error occurs during conversion, a ValueError is caught, and a message is displayed asking for a valid age. This loop continues until a valid age is successfully entered.
By implementing this improvement, the program becomes more resilient to unexpected inputs, providing a smoother and more user-friendly experience.
Handling user input errors is a crucial aspect of programming, ensuring that applications remain robust and user-centric. By incorporating error-handling mechanisms, developers can create more reliable and user-friendly software. Python’s versatility allows for such improvements, enhancing the overall user experience.
In conclusion, by addressing the handling of unexpected user input errors, developers can significantly improve the stability and usability of their programs. Embracing these techniques can lead to more resilient and user-friendly applications, making the interaction between software and users more efficient and pleasant.